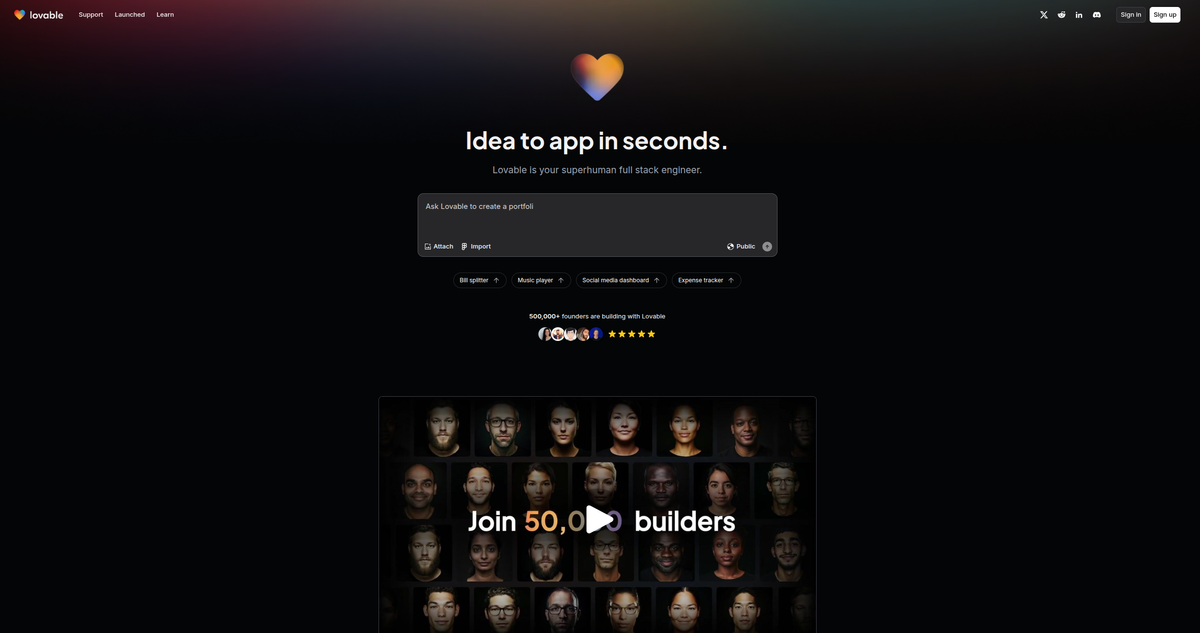
Conversational Coding for a React + Supabase URL Shortener
Lovable is an AI-first coding assistant platform that lets you build entire applications through natural language conversation. Designed to feel like pair programming with an intuitive and responsive agent, Lovable emphasizes simplicity, speed, and creativity. Unlike traditional IDEs, its core interface is a single chat where you describe your intent—and the system builds the scaffolding, UI, and logic for you.
It supports both frontend and backend frameworks, with seamless integration for popular tools like Supabase, making it ideal for solo developers, rapid prototyping, or exploring new ideas. Whether you're a beginner or a seasoned dev, Lovable offers a minimalist environment that keeps you focused on flow, not configuration.
Ok, let's start to built a URL shortener using React and Supabase on Lovable. Originally, I planned to build the app with a React frontend, a NestJS backend, and MongoDB. However, after working through multiple iterations with Lovable, I realized its Supabase integration was smoother and better supported. I eventually dropped the backend and MongoDB altogether in favor of a Supabase-powered frontend-only approach.
I kicked off the project with this prompt:
Prompt
I want you to act as an expert full-stack developer and help me build a URL shortener web app with usage analytics functionality. The project should be divided into three parts: a frontend in ReactJS, a backend in NestJS, and a MongoDB database.
Main Functionality:
- The user can input a long URL and the system will automatically generate a short URL.
- When a short URL is visited, the system redirects the user to the original URL.
- Generated URLs must be unique and persistent.
- Users should be able to easily copy the shortened URL from the frontend.
- There must be validation to ensure the entered URL is valid.
- Optionally, allow custom aliases if they are not already in use.
Usage Analytics Features:
- Each time a short URL is accessed, record:
- Timestamp of the click
- Visitor's IP address
- User-agent string
- Country (retrieved via GeoIP API)
- Total number of clicks per URL
- On the frontend, display a panel with analytics for each shortened URL:
- Visits per day chart
- Country distribution
- Total number of visits
- Most recent accesses
Frontend Requirements (ReactJS):
- Homepage with a form to shorten URLs
- Component to list the user's shortened URLs (login can be simulated)
- Dashboard to view analytics for a specific URL
- Good UI/UX design (use Tailwind or Material UI)
- Connect to the backend via REST API (Axios or Fetch)
Backend Requirements (NestJS):
- Endpoint to create a short URL from a long one
- Endpoint to handle redirection from the short URL
- Endpoint to record each access with analytics data
- Endpoint to retrieve detailed statistics for a URL
- Input validation, error handling, and data sanitization
- Store all information in MongoDB
Database Requirements (MongoDB):
urls
collection with fields:_id
,originalUrl
,shortCode
,createdAt
,clicks
,customAlias?
analytics
collection with fields:_id
,shortCode
,timestamp
,ip
,userAgent
,country
Generate a recommended folder structure for both frontend and backend, and provide minimal code examples for each core feature. Also include recommendations for implementing IP geolocation and best practices for generating unique short codes.
The result? A functional app in a couple of days, mostly driven by the AI assistant while I worked on other tasks in parallel.
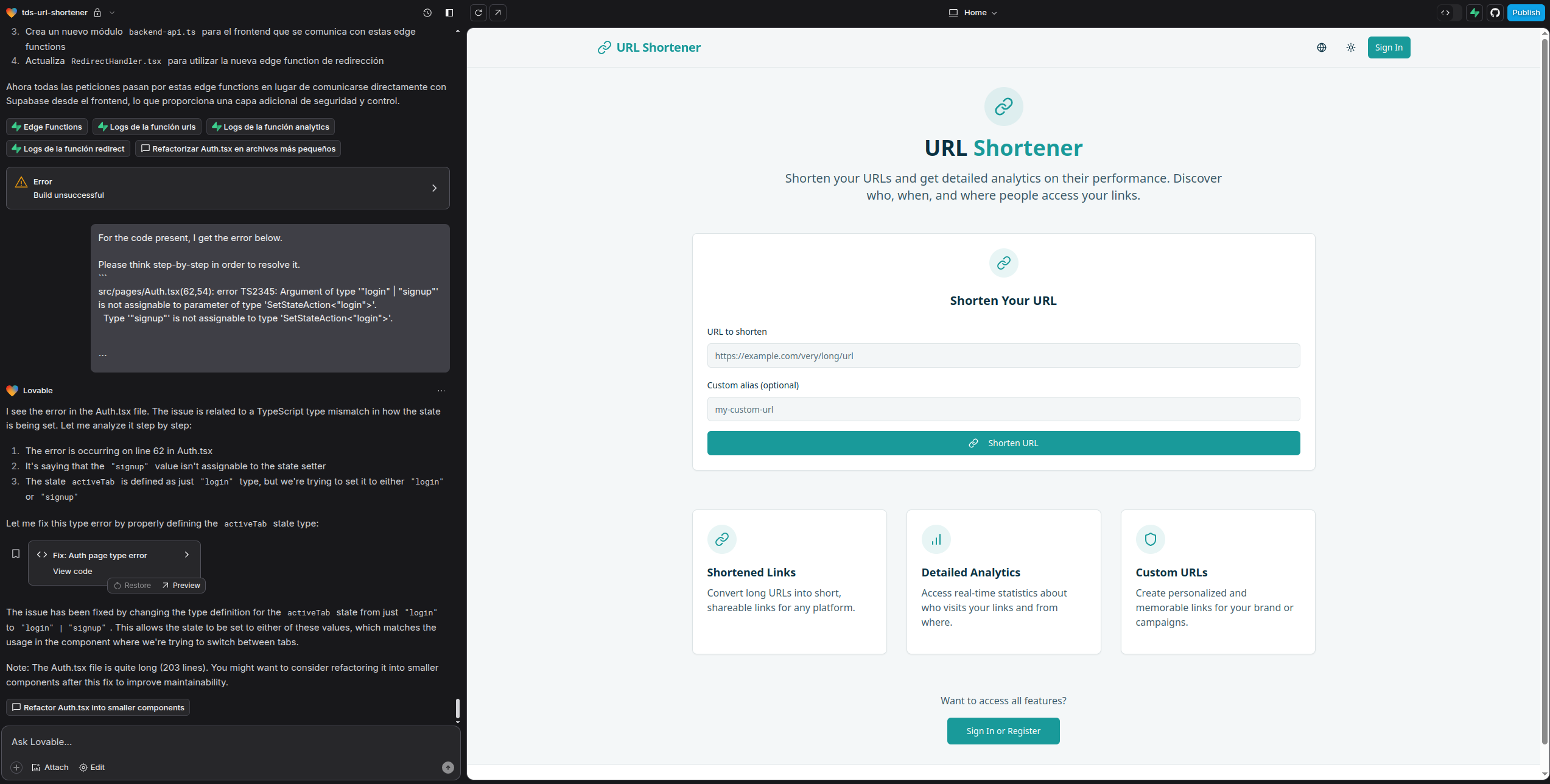
You can also see the progress in GitHub.
Summary Table
Category | Observations |
---|---|
Highlights | • Super simple UI—just a chat interface • Well-structured code output • Excellent for quick builds, especially small tools or MVPs |
Pain Points | • Daily limit of interactions can block your flow • For more complex issues, you’ll have to dive into the code yourself |
Lovable is an excellent entry point to vibe coding, especially for simpler tools or when you're looking to test new ideas fast.